Welcome, dear code newbie! If you’re here, it means you’ve finally mustered the courage to learn C++, the most needlessly complex and arcane programming language ever devised. Don’t worry, we’ll walk you through it, slowly and gently, like a toddler learning to use the potty.
With this complete guide, you’ll go from coding virgin to full-blown C++ guru. Just buckle up, chug a Red Bull, and get ready for the ride of your life. Before you know it, you’ll be cranking out linked lists and binary trees like a boss. So grab your pocket protector, tape up those glasses, and let’s do this!
How to Learn C++ Programming as a Beginner
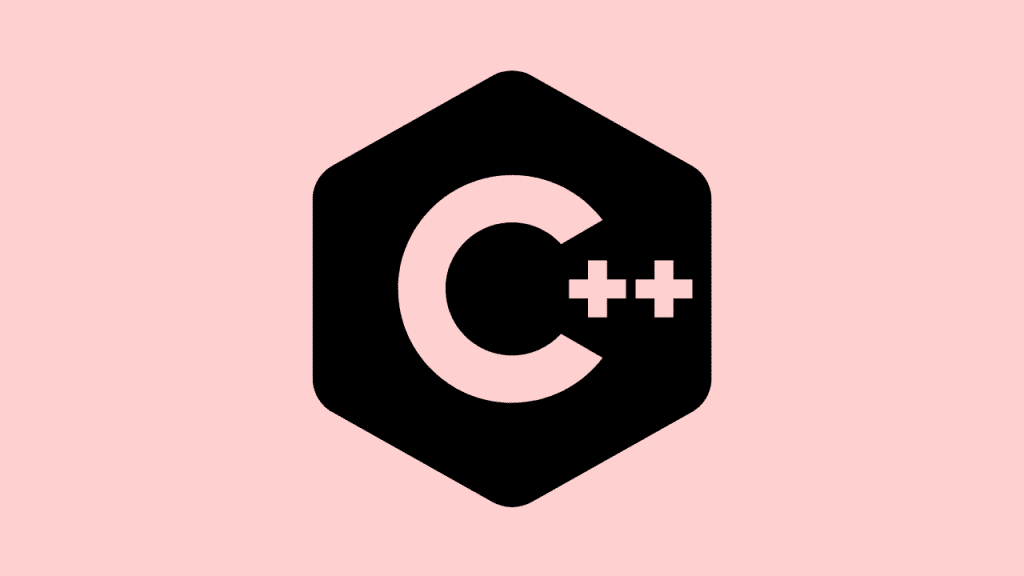
So you want to learn C++, do you? Brace yourself for a long, frustrating road ahead. The first step is to find some good learning resources. You’ll have no shortage of options here – books, online courses, video tutorials.
Whatever medium suits your fancy. The key is to start with the basics. Learn what a variable is, how functions work, the difference between ints and doubles. Thrilling stuff, right?
Don’t Get Discouraged
Once you’ve grasped the fundamentals, it’s time to start building simple programs. A prime number checker. A basic calculator. It’s a guessing game. These help you get a feel for actually using the language. But here’s a warning: your first programs will be ugly. You’ll look at the code of experienced programmers and think you’ll never reach their level.
Practice Every Day
The only way to get better is to practice. Try to code every day, even if just for 30 minutes. Over time, those hours add up. You’ll get more comfortable with syntax, start thinking logically in C++, and your skills will improve. Before you know it, you’ll be ready to tackle more complex programs. A text editor. A desktop app. A mobile game. The possibilities are endless!
Keep Learning
The final step is never to stop learning. C++ is a huge language with many nuances. Frameworks, libraries, and new standards are always emerging. Stay up-to-date with resources like cppreference.com and cplusplus.com.
Read books on advanced C++ concepts. Take additional online courses. The more you learn, the more you’ll realize how much you still don’t know. But that’s the fun part – there’s always more to discover in the world of C++!
Learn C++ Syntax and Variables
So you want to learn C++, do you? Well, you’ve got your work cut out for you. C++ is not for the faint of heart. To start, you’ll need to familiarize yourself with the basic C++ syntax. We’re talking variables, data types, operators, control flows – all that good stuff.
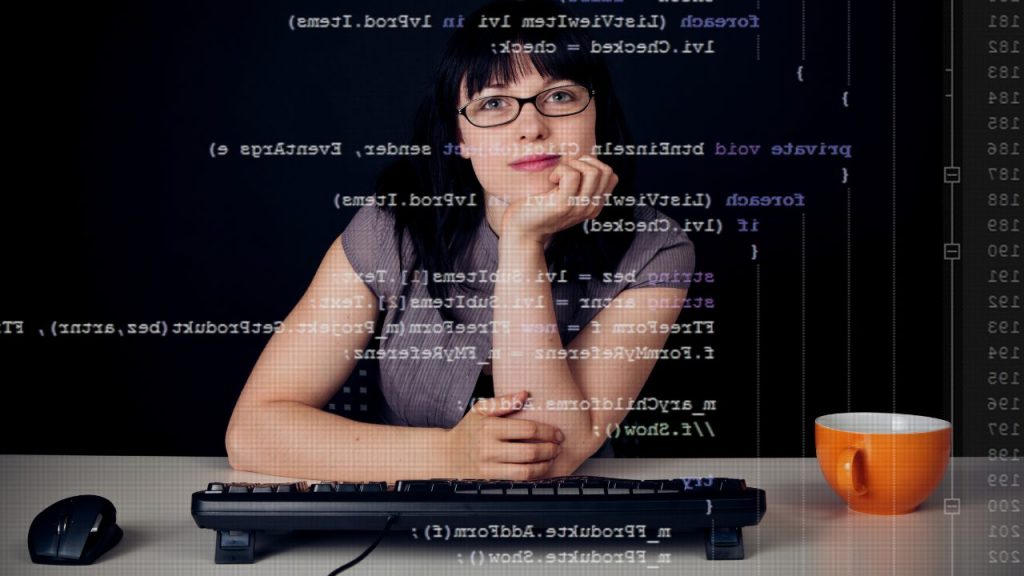
You’ll be spending a lot of quality time with variables, which are basically containers that hold data. In C++, you’ll have to specify a data type for each variable, like int for integers or std::string for strings. No wishy-washy type inference here! You’ll get familiar with C++’s built-in types like char, int, float, double, and bool.
Read More : Learn React JS for Free: Complete React Roadmap in 2024
Use Meaningful Variable Names
When naming your variables, be descriptive. No single-letter names allowed! Use full words that make sense, like age
instead of a
. Your future self will thank you. C++ is already confusing enough without having to decode obscure variable names.
Know Your Operators
You’ll also need to memorize C++’s operators, like + for addition, – for subtraction, * for multiplication, and / for division. But wait, there’s more! C++ has operators for incrementing ++, decrementing –, assignment =, comparison == and !=, and logical operators like && for AND and || for OR.
Control the Flow
To direct the flow of your programs, you’ll rely on control flows like if-else statements, for loops, and while loops. If you have experience with other languages, these will look familiar. If not, you’ve got some learning to do!
With the basics of C++ syntax under your belt, you’ll be ready to move on to more advanced topics. But take your time – C++ has a steep learning curve, and you’ll need a solid foundation to build upon. syntax and variables are just the beginning of your C++ journey!
Master C++ Loops, Arrays, and Functions
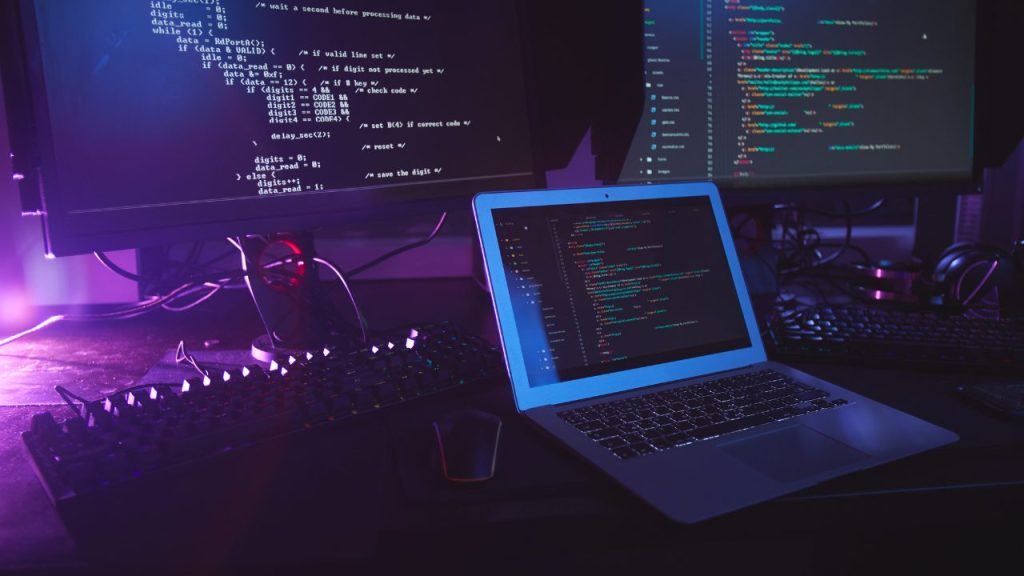
Loops: Repeating Yourself Without Going Insane
Loops are your new best friend for avoiding tedious repetition in your code. Use a for loop when you know how many times you want to repeat something, like:
for (int i = 0; i < 5; i++) {
std::cout << "Hello!";
}
This will print “Hello!” five times. If you don’t know how many times you need to loop in advance, a while loop is your guy:
int i = 1;
while (i <= 10) {
std::cout << i;
i++;
}
This will print the numbers 1 through 10. Just be sure to include a way to eventually exit the loop, or your program will be stuck in an infinite loop (awkward!).
Arrays: When One Variable Isn’t Enough
If you have a bunch of related data you want to store together, an array is your new BFF. You declare an array like this:
int ages[5] = {18, 22, 15, 27, 30};
This creates an array named ages
with 5 elements. You can access each element using bracket notation and the index:
ages[0] = 18; // First element
ages[1] = 22; // Second element
Arrays are great when you know how much data you need to store ahead of time. If not, you’ll want to use a vector instead.
Functions: Breaking Up the Party
As your programs get more complex, you’ll want to organize your code into reusable functions. A basic function looks like this:
void sayHello() {
std::cout << "Hello!";
}
You define a function using the void
keyword (for functions that don’t return a value), the function name, and parentheses ()
. The function body is enclosed in curly brackets {}
.
To call or execute the function, use its name followed by parentheses:
sayHello(); // Prints "Hello!"
Functions let you tidy up your code and avoid duplication. Use them liberally! Your future self will thank you.
Learn C++ by Example: A Simple Program Walkthrough
So you want to learn C++, do you? Brace yourself for a wild ride through pointers, references, classes, and memory management. But don’t worry, we’ll start simple. Every C++ program begins with #include <iostream>
, which gives you access to the input/output stream library.
The Mighty main()
Next up is int main()
, the function where all the magic happens. Whatever is in this function will run when you execute your program.
int main() {
// This is a comment! Comments are ignored by the compiler.
}
For now, our main function will remain empty. Compile and run this, and…nothing happens! How thrilling. Let’s have some real fun and add:
std::cout << "Hello World!";
This line prints “Hello World!” to the console. Compile and run again, and voila! Your first C++ program. Give yourself a pat on the back, you’re well on your way to becoming a coding wizard.
Variables
Of course, printing static text is pretty boring. We need variables! A variable holds a value that can change. You define a variable like this:
int myVariable = 10; // myVariable is an integer with value 10
Now we can print the variable:
std::cout << myVariable; // Prints 10
We can also change the variable value and print it again:
myVariable = 5;
std::cout << myVariable; // Now prints 5
Operators
C++ has all the usual operators you’d expect for manipulating variables:
int sum = 5 + 10; // Addition, sum is now 15
int product = 5 * 2; // Multiplication, product is 10
int difference = 10 - 5; // Subtraction, difference is 5
And with that, you’ve got the basics of variables, printing to the console, and math operators in C++. Not too shabby! Keep practicing and before you know it, you’ll be writing complex C++ programs and annoying your friends with explanations of pointers and references. Happy coding!
How to Learn C++ for Free Online
So you want to learn C++ but don’t want to drop major dough on expensive courses or degrees. No worries, the Interwebs have you covered. These days you can find everything from interactive tutorials to full courses online for the low, low price of zero dollars.
DIY with Interactive Tutorials
If you’re the self-motivated type, interactive tutorials are a great way to pick up C++ basics on your own schedule. Sites like LearnCpp.com and TutorialsPoint offer step-by-step tutorials to walk you through fundamentals like data types, operators, functions, and classes.
They’re simple but effective. Be warned though, you’re on your own here without an instructor to pester with questions. Hope you don’t get stuck in an infinite loop!
Take a Free Online Course
Massive open online courses or MOOCs offer more structure than DIY tutorials. Platforms like Coursera, Udacity, and edX offer free C++ courses where you’ll code along with instructors, complete assignments, and earn a certificate of completion.
The downside is courses follow a set schedule so you need to keep pace or get left behind. But at least there’s a community of other learners to commiserate with.
Watch Tutorial Videos
If reading tutorials sounds about as fun as watching paint dry, video tutorials may be more your style. Websites like Udemy, Pluralsight, and Udacity offer video-based C++ courses for beginners. Instructors walk through concepts and code line by line on video while you follow along.
The pacing is up to you – rewind and re-watch anytime. Though be warned, C++ isn’t the most riveting subject matter, even on video. Have coffee handy! The road to mastering C++ is long, but these free resources can help you pave the way.
With interactive tutorials, online courses, and video tutorials at your disposal, you have no excuse not to start learning C++ today. Just choose your own adventure and code away! Now if you’ll excuse me, I have a date with a compiler. Happy learning!
Read More : Flutter Developer Roadmap in 2024 : Jobs And Salary Guides
Best C++ Tutorials and Courses for Beginners
So, you want to learn C++, do you? Brace yourself, my friend, because you’re in for a wild ride. C++ is not for the faint of heart. It’ll scramble your brain like eggs and have you questioning your very existence. C++ can admittedly be challenging to pick up, but with the right resources, you’ll be cranking out “Hello, World!” apps in no time. Here are some of the best ways to get your feet wet with C++.
Tutorials for the DIY Learner
If you prefer to learn at your own pace for free, check out Learn C++, C++ Tutorial, and The C++ Tutorial. These handy tutorials walk you through C++ basics step-by-step with examples. You’ll start with fundamentals like data types, operators, and control flows before moving on to classes, objects, and inheritance.
Interactive Coding Websites
Sites like Codecademy, Datacamp, and Codewars let you write and run C++ code right in the browser. You’ll get instant feedback as you solve interactive exercises and challenges. These “learn by doing” platforms are perfect for beginners, but the lessons can feel a bit superficial. You’ll still need to dive into meatier resources to become truly proficient.
Video Courses
Maybe you’re more of a visual learner. In that case, video courses on Udemy, Coursera, and Udacity are a great option. Instructors walk you through setting up your environment, basic syntax, and project ideas. Some courses even offer mentor support and code reviews. While not always free, video courses provide an interactive learning experience that’s as close to in-person instruction as you can get.
The path to C++ mastery is long, but with patience and perseverance, you’ll get there. And once you do, you’ll have a powerful new skill that opens you up to a world of opportunities. Now get crackin’! Hello World apps are waiting to be written.
Learn C++ for Game Development
Do you want to make video games? Join the club, kid. Every wannabe programmer under the sun has dreamed of crafting the next blockbuster. The good news is, that with C++ under your belt, you’ll be well on your way to doing just that.
Pick an Engine, Any Engine
The major game engines like Unreal and Unity all use C++, so you’ll fit right in. Once you get the hang of the language, you can start building prototypes and dabbling in engine development. Who knows, you might craft the next big thing in gaming and become an overnight billionaire. Stranger things have happened.
Math Mania
Any game dev worth their salt has to know math, and I’m not talking just addition and subtraction. We’re talking linear algebra, trigonometry, and calculus. Don’t worry, you don’t need a PhD, but you’ll want a solid understanding of vectors, matrices, and how to calculate the trajectory of a projectile. C++ has libraries to help with the heavy lifting, but you’ve got to know what’s going on under the hood.
Physics for Fun and Profit
Once you’ve got the math down, it’s time to make things move. You’ll use your C++ skills to implement collisions, simulate gravity and friction, and make objects interact realistically. Ragdoll physics, anyone? If you can get this right, your games will go from amateur hour to pro level like that.
The Details, Man, the Details
The biggest challenge in game dev is handling all the minutiae – assets, animations, shaders, lighting, audio, UI, controls, the list goes on. Don’t worry, you’ve got this – with patience and persistence, you can work through any challenge. Think of it like a quest in one of your favorite games. There are a million little tasks, but achieving victory will be oh-so-sweet!
So there you have it, the roadmap to game dev glory. Learn C++, pick an engine, math it up, physics the heck out of it, and handle the details. With enough dedication, in no time you’ll be crafting your own virtual worlds for people to explore. Now get to work, you’ve got games to build!
Additional C++ Learning Resources
So, you’ve decided to embark on the fantastical journey that is learning C++. Mazel tov! Now comes the not-so-fun part of finding good resources for learning this beast of a programming language. While there’s no shortage of books, video courses, and interactive tutorials out there for learning C++, not all are created equal.
Some will have you bored to tears in a matter of minutes, with dry, overly technical explanations that suck the joy out of coding. Others are filled with so many jokes and pop culture references that you can’t remember if you actually learned anything.
What you need are resources that strike a balance between entertaining and educational. Resources with instructors who can inject humor and levity without distracting from the actual learning. Resources that provide real-world examples and projects so you can start building cool stuff right away, rather than just learning syntax.
A few recommendations:
- Udemy has some highly-rated video courses for learning C++. Look for ones from instructors like Kate Gregory, a C++ expert, and all-around programming badass. Her courses are technical yet fun, with real projects to work on.
- Coursera offers interactive C++ courses where you can code right in the browser. Some are more beginner-focused, others more advanced. The interactive exercises and quizzes are helpful for reinforcing concepts.
- Check out “C++ Crash Course” by Josh Lospinoso. This book provides a fast-paced but thorough overview of the C++ programming language. Written in a simple, straightforward style with humor and references to internet culture. Great for beginners.
- “The C++ Programming Language” by Bjarne Stroustrup, the creator of C++, is a classic reference book. A bit dense but filled with valuable insights into C++. Not for beginners but helpful as you advance.
- Follow C++ experts on YouTube, like The Cherno and Jason Turner. Their tutorial videos cover both C++ basics as well as more advanced topics. Entertaining but still focused, with lots of code examples.
With resources like these, you’ll be coding in C++ and building your own programs in no time. Best of luck, and happy learning!
Conclusion
And there you have it, my aspiring code monkey. The complete roadmap to learning C++, from installing your first compiler to building complex programs. Was it easy? Heck no. Did we have to slog through some seriously boring concepts along the way? You betcha. But now you’re armed with the power and versatility of C++.
Go forth and build that world-changing app, or just make your computer flash pretty colors on the screen. The programming world is your oyster. Just try not to crash the whole thing, okay? We’re all counting on you, C++ newbie. Now get out there and #include <iostream> like you mean it.
Read More : Programming Languages for Data Analysis and Visualization in 2024